clrscr() and getch() both are predefined function in "conio.h" (console input output header file).
Clrscr()
It is a predefined function in "conio.h" (console input output header file) used to clear the console screen. It is a predefined function, by using this function we can clear the data from console (Monitor). Using of clrscr() is always optional but it should be place after variable or function declaration only.
Example of clrscr()
#include<stdio.h> #include<conio.h> void main() { int a=10, b=20; int sum=0; clrscr(); // use clrscr() after variable declaration sum=a+b; printf("Sum: %d",sum); getch(); }
Output
Sum: 30
Getch()
It is a predefined function in "conio.h" (console input output header file) will tell to the console wait for some time until a key is hit given after running of program.
By using this function we can read a character directly from the keyboard. Generally getch() are placing at end of the program after printing the output on screen.
Example of getch()
#include<stdio.h> #include<conio.h> void main() { int a=10, b=20; int sum=0; clrscr(); sum=a+b; printf("Sum: %d",sum); getch(); // use getch() befor end of main() }
Output
Sum: 30
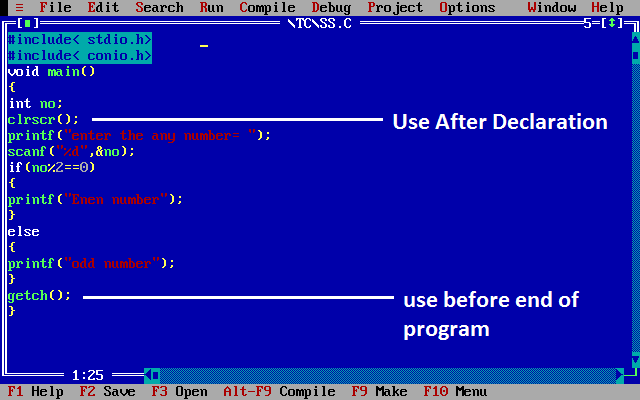
Read and Write Character in C
We can read and write a character on screen using printf() and scanf() function but this is not applicable in all situations. In C programming language some function are available which is directly read a character or number of character from keyboard.
getchar
This is a predefined function in C language which is available in stdio.h header file. Using this function we can read a single character from keyboard and store in character variable. When we want to read a number of character form keyboard the store all the data in a character array.
Example
char ch; ch=getch();
Note: getchar function has no any parameters.
gets
This is a predefined function in C language which is available in stdio.h header file. This function is used to read a single string from keyboard.
Example
gets(string);
putchar
putchar function is a same as getchar but is function is used for display a character value of screen or console. This function must be take one parameters.
Example
char ch='A'; putchar (ch);
putchar is equivalent to printf("%c",ch);
puts
puts is same as gets function but this is used to display a string on screen or console. This function takes single arguments.
Example
puts(str);
No comments:
Post a Comment