Typedef
The C programming language provides a keyword called typedef, by using this keyword you can create a user defined name for existing data type. Generally typedef are use to create an alias name (nickname).
Declaration of typedef
Syntax
typedef datatype alias_name;
Example
typedef int Intdata;
Example of typedef
#include<stdio.h> #include<conio.h> typedef int Intdata; // Intdata is alias name of int void main() { int a=10; Integerdata b=20; typedef Intdata Integerdata; // Integerdata is again alias name of Intdata Integerdata s; clrscr(); s=a+b; printf("\n Sum:= %d",s); getch(); }
Output
Sum: 20
Code Explanation
- In above program Intdata is an user defined name or alias name for an integer data type.
- All properties of the integer will be applied on Intdata also.
- Integerdata is an alias name to existing user defined name called Intdata.
Note: By using typedef only we can create the alias name and it is under control of compiler.
You can in another example, here myint is alias name for integer data and again smallint is alias name for myint.
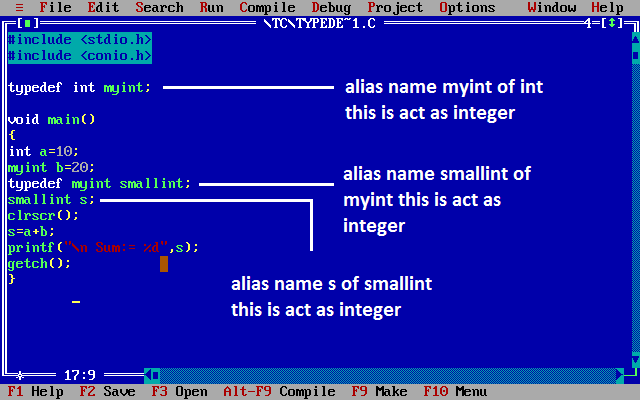
Buffer in C
Temporary storage area is called buffer.
All standard input output devices are containing input output buffer.
In implementation when we are passing more than required number of values as a input then rest of all values will automatically holds in standard input buffer, this buffer data will automatically pass to next input functionality if it is exist.
Example
#include<stdio.h> #include<conio.h> void main() { int v1,v2; clrscr(); printf("\n Enter v1 value: "); scanf("%d",&v1); printf("\n Enter v2 value: "); scanf("%d",&v2); printf("\n v1+v2=%d ",v1+v2); getch(); }
Output
Enter v1 value: 10 Enter v2 value: 20 V1+v2=30 Again run program Enter v1 value: 10 20 30 Enter v2 value: V1+v2=30
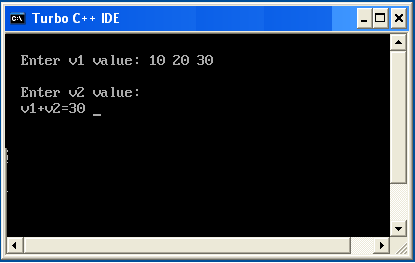
Explanation:
In the above example we pass three input in v1 and we cannot pass any value in v2 but value of v1 is automatically pass in v2.
In implementation when we need to remove standard input buffer data then go for flushall() or fflush() function.
flushall()
it is a predefined function which is declared in stdio.h. by using flushall we can remove the data from standard input output buffer.
fflush()
it is a predefined function in "stdio.h" header file used to flush or clear either input or output buffer memory.
fflush(stdin)
it is used to clear the input buffer memory. It is recommended to use before writing scanf statement.
fflush(stdout)
it is used to clear the output buffer memory. It is recommended to use before printf statement.
Example
#include<stdio.h> #include<conio.h> void main() { int v1,v2; clrscr(); printf("\n Enter v1 value: "); scanf("%d",&v1); printf("\n Enter v2 value: "); fflush(stdin); scanf("%d",&v2); printf("\n v1+v2=%d ",v1+v2); getch(); }
Output
Enter v1 value: 10 20 30 Enter v2 value: 40 v1+v2=50
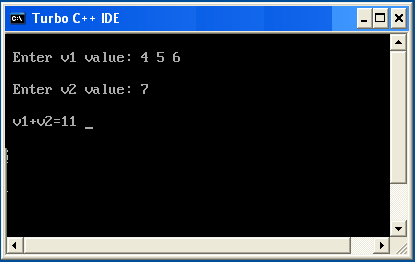
Pre-processor in C
Preprocessor is a program which will executed automatically before passing the source program to compiler. This process is called pre-processing. The preprocessor provides the ability for the inclusion of header files, macro expansions, conditional compilation, and line control.
The C Preprocessor is not a part of compiler, it is a separate program invoked by the compiler as the first part of translation.
Commands used in preprocessor are called preprocessor directives and they begin with pond "#" symbol and should be not ended with (;).
Defined Proprocessor Directive
Proprocessor Directive can be place any where in the program, but generally it place top of the program before defining the first function.
Example
#include<stdio.h> #define PI 3.14 void main() { printf("%f",PI); }
Output
3.14
C language preprocessor directives
- Macro substitution directives. example: #define
- File inclusion directives. example: #include
- Conditional compilation directive. example: #if, #else, #ifdef, #undef
- Miscellaneous directive. example: #error, #line
Preprocessor | Syntax | Description |
---|---|---|
Macro | #define | This macro defines constant value and can be any of the basic data types. |
Header file inclusion | #include <file_name> | The source code of the file "file_name" is included in the main program at the specified place |
Conditional compilation | #ifdef, #endif, #if, #else, #ifndef | Set of commands are included or excluded in source program before compilation with respect to the condition |
Other directives | #undef, #pragma | #undef is used to undefine a defined macro variable. #Pragma is used to call a function before and after main function in a C program |
#ifdef, #else and #endif
"#ifdef" directive checks whether particular macro is defined or not. If it is defined, "If" clause statements are included in source file. Otherwise, "else" clause statements are included in source file for compilation and execution.
Example
#include<stdio.h> #include<conio.h> #define AGE 18 void main() { clrscr(); #ifdef AGE { printf("Eligible for voting\n"); } #else { printf("Not eligible\n"); } #endif getch(); }
Output
Eligible for voting
#if, #else and #endif
"If" clause statement is included in source file if given condition is true. Otherwise, else clause statement is included in source file for compilation and execution.
Example
#include<stdio.h> #include<conio.h> #define AGE 18 void main() { clrscr(); #if (AGE>=18) { printf("Eligible for voting"); } #else { printf("\nNot eligible"); } #endif getch(); }
Output
Eliginle for voting
undef
This directive undefines existing macro in the program. In below program we first undefine age variable and again it define with new value.
Example
#include<stdio.h> #include<conio.h> #define age 18 void main() { clrscr(); printf("First defined value for age: %d\n",age); #undef age // undefining variable #define age 30 // redefining age value for for new value printf("Age after undef redefine: %d",age); getch(); }
Output
First defined value for age: 18 Age after undef redefine: 30
pragma
Pragma is used to call a function before and after main function in a C program.
Example
#include<stdio.h> #include<conio.h> #include<dos.h> void function1( ); void function2( ); #pragma startup function1 #pragma exit function2 void main( ) { delay(1000); printf ("\nI am main function" ) ; } void function1( ) { clrscr(); delay(500); printf("\nI am function1"); } void function2( ) { delay(1000); printf ( "\nI am function2" ); getch(); }
Output
I am function1 I am main function I am function2
Explanation: Here delay() function are used for give delay or wait time for execution of code, this function is present in dos.h header file. With the help of delay function you can see clearly flow of above program.
Header Files in C
Header files contain definitions of functions and variables, which is imported or used into any C program by using the pre-processor #include statement. Header file have an extension ".h" which contains C function declaration and macro definition.
Each header file contains information (or declarations) for a particular group of functions. Like stdio.h header file contains declarations of standard input and output functions available in C which is used for get the input and print the output. Similarly, the header file math.h contains declarations of mathematical functions available in C.
Types of Header Files in C
- System Header Files: It is comes with compiler.
- User header files: It is written by programmer.
Why need of header files
When we want to use any function in our c program then first we need to import their definition from c library, for importing their declaration and definition we need to include header file in program by using #include. Header file include at the top of any C program.
For example if we use printf() in C program, then we need to include, stdio.h header file, because in stdio.h header file definition of printf() (for print message on screen) is written in stdio.h header file.
Syntax Header Files in C
#include<stdio.h>
How to use Header File in Program
Both user and system header files are include using the pre-processing directive #include. It has following two forms:
Syntax
#include<file>
This form is used for system header files. It searches for a file named file in a standard list of system directives.
Syntax
#include"file"
This form used for header files of our own program. It searches for a file named file in the directive containing the current file.
Note: The use of angle brackets <> informs the compiler to search the compilers include directory for the specified file. The use of the double quotes "" around the filename inform the compiler to search in the current directory for the specified file.
Compile and Link C Program
There are three basic phases occurred when we execute any C program.
- Preprocessing
- Compiling
- Linking

Preprocessing Phase
A C pre-processor is a program that accepts C code with preprocessing statements and produces a pure form of C code that contains no preprocessing statements (like #include).
Compilation Phase
The C compiler accepts a preprocessed output file from the preprocessor and produces a special file called an object file. Object file contains machine code generated from the program
Linking Phase
The link phase is implemented by the linker. The linker is a process that accepts as input object files and libraries to produce the final executable program.
Dynamic Memory Allocation
- It is a process of allocating or de-allocating the memory at run time it is called as dynamically memory allocation.
- When we are working with array or string static memory allocation will be take place that is compile time memory management.
- When we ate allocating the memory at compile we cannot extend the memory at run time, if it is not sufficient.
- By using compile time memory management we cannot utilize the memory properly
- In implementation when we need to utilize the memory more efficiently then go for dynamic memory allocation.
- By using dynamic memory allocation whenever we want which type we want or how much we type that time and size and that we much create dynamically.
Dynamic memory allocation related all predefined functions are declared in following header files.
- <alloc.h>
- <malloc.h>
- <mem.h>
- <stdlib.h>
Dynamic memory allocation related functions
Malloc()
By using malloc() we can create the memory dynamically at initial stage. Malloc() required one argument of type size type that is data type size malloc() will creates the memory in bytes format. Malloc() through created memory initial value is garbage.
Syntax:
Void*malloc(size type);
Note: Dynamic memory allocation related function can be applied for any data type that's why dynamic memory allocation related functions return void*.
When we are working with dynamic memory allocation type specification will be available at the time of execution that's why we required to use type casting process.
Syntax
int *ptr; ptr=(int*)malloc(sizeof (int)); //2 byte long double*ldptr; ldptr=(long double*)malloc(sizeof(long double)) // 2 byte char*cptr; cptr=(char*)malloc(sizeof(char)); //1 byte int*arr; arr=(int*)malloc(sizeof int()*10); //20 byte cahr*str; str=(char*)malloc(sizeof(char)*50); //50 byte
calloc()
- By using calloc() we can create the memory dynamically at initial stage.
- calloc() required 2 arguments of type count, size-type.
- Count will provide number of elements; size-type is data type size.
- calloc() will creates the memory in blocks format.
- Initial value of the memory is zero.
Syntax
int*arr; arr=(int*)calloc(10, sizeof(int)); // 20 byte cahr*str; str=(char*)calloc(50, siceof(char)); // 50 byte
realloc()
- By using realloc() we can create the memory dynamically at middle stage.
- Generally by using realloc() we can reallocation the memory.
- Realloc() required 2 arguments of type void*, size_type.
- Void* will indicates previous block base address, size-type is data type size.
- Realloc() will creates the memory in bytes format and initial value is garbage.
Syntax
void*realloc(void*, size-type); int *arr; arr=(int*)calloc(5, sizeof(int)); ..... ........ .... arr=(int*)realloc(arr,sizeof(int)*10);
free()
- When we are working with dynamic memory allocation memory will created in heap area of data segment.
- When we are working with dynamic memory allocation related memory it is a permanent memory if we are not de-allocated that's why when we are working with dynamic memory allocation related program, then always recommended to deleted the memory at the end of the program.
- By using free(0 dynamic allocation memory can be de-allocated.
- free() requires one arguments of type void*.
Syntax
void free(voie*); int *arr; arr=(int*)calloc(10,sizeof(int)); ... ...... free(arr);
- By using malloc(), calloc(), realloc() we can create maximum of 64kb data only.
- In implementation when we need to create more than 64kb data then go for formalloc(), forcalloc() and forrealloc().
- By using free() we can de-allocate 64kb data only, if we need to de-allocate more than 64kb data then go for
Syntax
forfree(). formalloc() voidfor*formalloc(size-type);
No comments:
Post a Comment